One of the best decisions we made at Coderockr was to adopt the "API First" approach for all the projects we started since 2010. However, in recent months, we recognized a need to improve the process of defining and documenting APIs.
We were already using other approaches , but most of them involved documenting the API within the code itself, using annotations. This approach works, but it has some issues, especially when documentation needs to be altered by someone in business. Additionally, generating mocks and tests from these annotations is a complex challenge.
With this in mind, we conducted some research and arrived at two alternatives: Swagger and API Blueprint. Both are API documentation standards and have their advantages and disadvantages:
Swagger: It is the market standard and has been adopted by several companies like Amazon. To describe the API, it is necessary to create JSON files, which greatly facilitates the process for programmers but is a bit complex for non-technical individuals to visualize and modify the content. There is a series of tools that can help with this process, but it has become a minor barrier for us. (Well, at least it's not YML... Did I mention that I hate YML?)
API Blueprint: This is a more recent specification created by a company called apiary , which was acquired by Oracle. The major advantage of API Blueprint is that it is written in Markdown, making it much easier to edit the documents, even for those who are not familiar with coding. Additionally, there are several tools available that allow the generation of documents in the Swagger format, mock servers, and tests.
We opted for API Blueprint because of its ease of use and the agility it brought us. I will demonstrate with a small example.
The definition is written in a file in Markdown format, which can be named “api.md” or “api.apib.” Both work, but if we use the ".apib" extension, we can take advantage of plugins for editors like Sublime Text that assist in writing. The plugins can be found on the official specification website.
On the specification website, you can see the details, but basically, what we do is define the URLs, the format of requests and responses. We can define simple and complex data structures and use them to describe what the API expects as input and what it should generate as output. The document is relatively simple to understand and modify, which was one of the key points in our choice. Even so, we can enhance the presentation.
Generating documentation
Among the tools available on the official website, aglio is one of the most interesting for generating an HTML presentation of our definition. It can be installed via:
To generate the documentation, we can use the command:
On the tool's website, you can see all the options for theme and presentation customization. Another useful command is:
It generates a local server on port 3000, which monitors changes in the ".apib" file and automatically updates the documentation page. This greatly facilitates the maintenance of the document. Here's an example of the generated documentation.
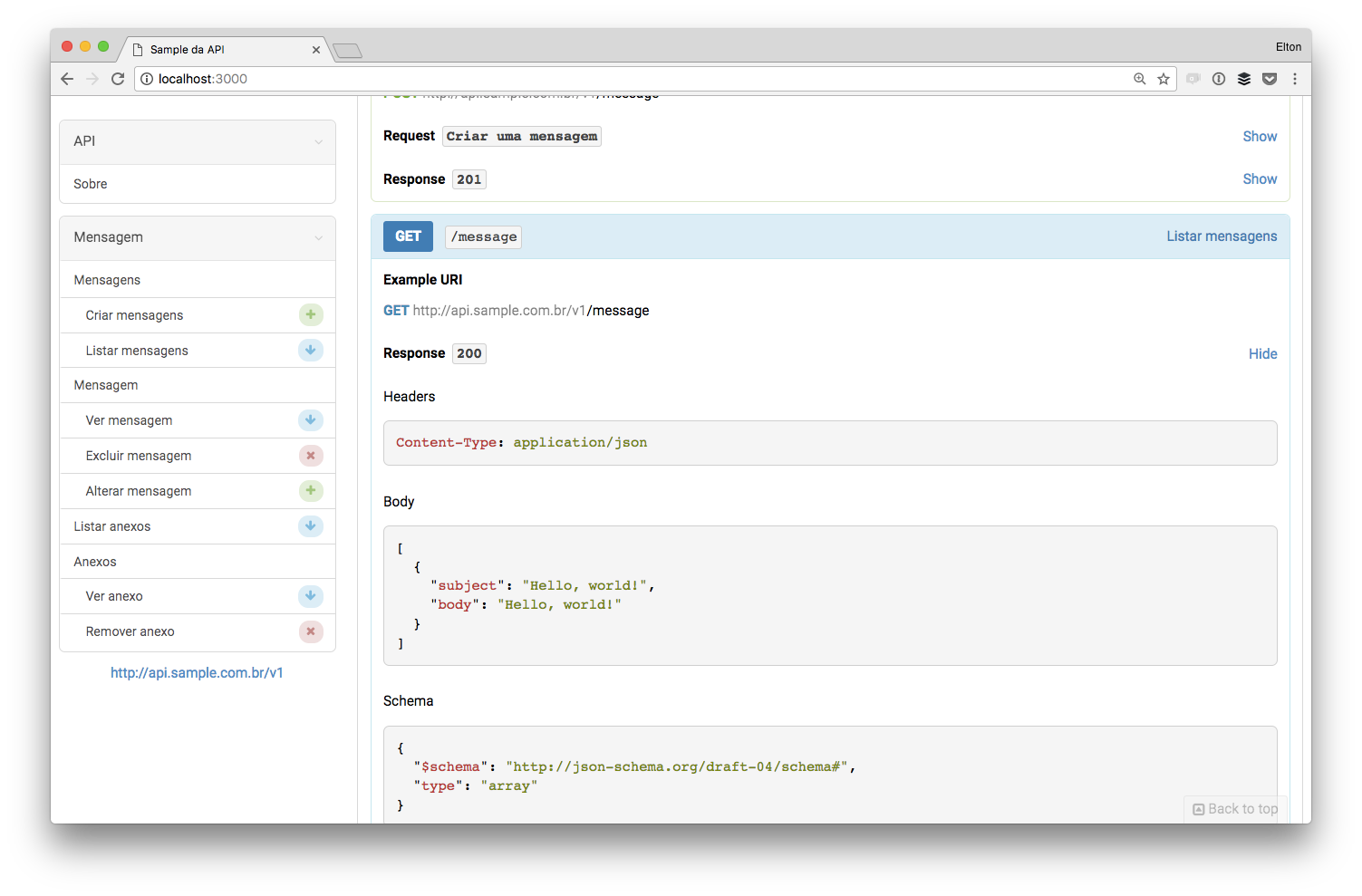
Documentation is very helpful in the development process for API clients, but we can go further.
Generating a mock server
With the API defined, frontend teams (web, mobile, etc.) and backend teams (who will develop the API) can work in parallel. To make this even easier, we can create a "mock server" that generates fake data based on the API definition. This way, the frontend team can work without having to wait for the backend team to finish the implementation. For this, we will use another tool, drakov .
To install the tool, simply run:
And to generate the server, run:
This way, we have a functional API that can be used for testing and development.
The final step is to define a way to validate our API.
Testing
We can use a tool called apib2swagger to generate a Swagger file for our API and perform tests using some of Swagger's features. We chose to use dredd , which automates tests using both API Blueprint and Swagger.
To install it, run:
And to run the tests, use the command:
In this example, I'm using dredd to test our "mock server," so the result should be positive. We can integrate Dredd into our continuous integration server to ensure that the API implementation always aligns with the documentation, avoiding surprises and abandoned documents.
With the combination of API Blueprint + aglio + drakov + dredd, we can map the entire lifecycle of an API: definition, documentation, development, and testing. The results have been very positive, and we should adopt this solution in all new projects.